nodejs와 selenium-webdriver에서 사용할 수 있는 Element에 대해 알아보겠습니다.
Element를 적절히 활용하면 selenium을 활용한 크롤링 혹은 웹 자동화를 할 수있습니다.
Element를 찾고 Element에서 제공하는 다양한 함수에 대해 알아보겠습니다.
예제에서는 chrome 브라우저를 사용합니다.
selenium WebElement nodejs
WebElement는 DOM element를 나타냅니다.
element를 찾을 떄는 Webdriver를 이용해서 찾을 수도 있고 다른 WebElement를 통해서도 찾을 수 있습니다.
const webdriver = require('selenium-webdriver');
const { By } = require('selenium-webdriver');
const chrome = require('selenium-webdriver/chrome');
const run = async () => {
const service = new chrome.ServiceBuilder('./chromedriver').build();
chrome.setDefaultService(service);
const driver = await new webdriver.Builder()
.forBrowser('chrome')
.build();
await driver.get('https://www.tistory.com/');
const info_div = await driver.findElement(By.className('btn_tistory '));
start_btn.click();
setTimeout(async () => {
await driver.quit();
process.exit(0);
}, 3000);
}
run();
selenium findElement, findElements nodejs
findElement
: 일치하는 하나의 Element를 반환합니다.
findElements
: 일치하는 모든 Elements를 array
로 반환합니다.
findElement
await driver.get('https://www.tistory.com/');
const startBtn = await driver.findElement(By.css('#kakaoHead > div > div.info_tistory > div > a'));
console.log(await startBtn.getText());
findElements nodejs
await driver.get('https://www.tistory.com/');
const gnbMenus = await driver.findElements(By.css('#kakaoGnb > ul > li'));
console.log(gnbMenus.length);
console.log(await gnbMenus[0].getText());
findElements는 array를 리턴합니다.
selenium sendKeys nodejs
문자 데이터를 입력하거나 Key 입력 이벤트를 발생시킬 수 있습니다.
const { By, Key } = require('selenium-webdriver');
await driver.get('https://www.tistory.com/auth/login/old?redirectUrl=https%3A%2F%2Fwww.tistory.com%2F');
const loginInput = await driver.findElement(By.css('#loginId'));
await loginInput.sendKeys("email", "abc")
const pwInput = await driver.findElement(By.css('#loginPw'));
await pwInput.sendKeys("password", Key.ENTER)
Key.ENTER를 사용하여 패스워드 입력 후 엔터를 입력합니다.
selenium element click nodejs
element의 click 이벤트를 발생시킵니다.
await driver.get('https://www.tistory.com/auth/login/old?redirectUrl=https%3A%2F%2Fwww.tistory.com%2F');
const loginInput = await driver.findElement(By.css('#loginId'));
await loginInput.sendKeys("email", "abc")
const pwInput = await driver.findElement(By.css('#loginPw'));
await pwInput.sendKeys("password")
const loginBtn = await driver.findElement(By.css('#authForm > fieldset > button'));
await loginBtn.click();
로그인 버튼 element를 찾고 click() 함수를 이용하여 click이벤트를 발생시킵니다.
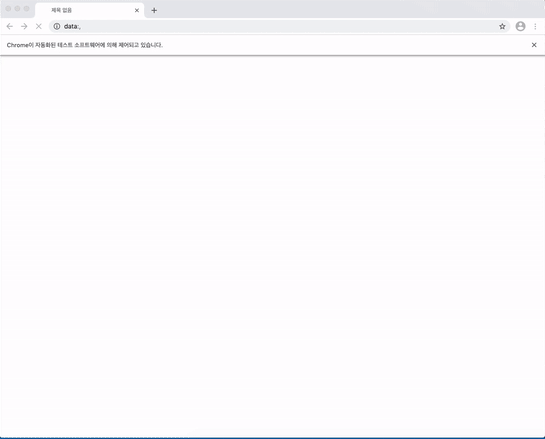
selenium element getText nodejs
element의 sub-elements 포함한 모든 텍스트를 return합니다.
await driver.get('https://www.tistory.com/auth/login/old?redirectUrl=https%3A%2F%2Fwww.tistory.com%2F');
const fields = await driver.findElement(By.css('#authForm > fieldset'));
console.log(await fields.getText());
// output
// 로그인
// 로그인 상태 유지
// 아이디 / 비밀번호 찾기
element를 활용하여 원하는 DOM을 찾거나 혹은 모든 DOM 리스트를 찾는 방법에 대해 알아보겠습니다.
다음에는 By를 활용한 DOM을 찾는 다양한 방법에 대해 알아보겠습니다.
[Selenium-크롤링] - selenium 크롤링 nodejs - 브라우저 열기
[Selenium-크롤링] - selenium element selector By nodejs
'Selenium' 카테고리의 다른 글
selenium element timeout/wait By nodejs (0) | 2021.01.18 |
---|---|
selenium WebElement python (0) | 2021.01.14 |
selenium python 페이지 열기 (0) | 2021.01.13 |
selenium element selector By nodejs (0) | 2021.01.12 |
selenium 크롤링 nodejs - 브라우저 열기 (3) | 2021.01.11 |